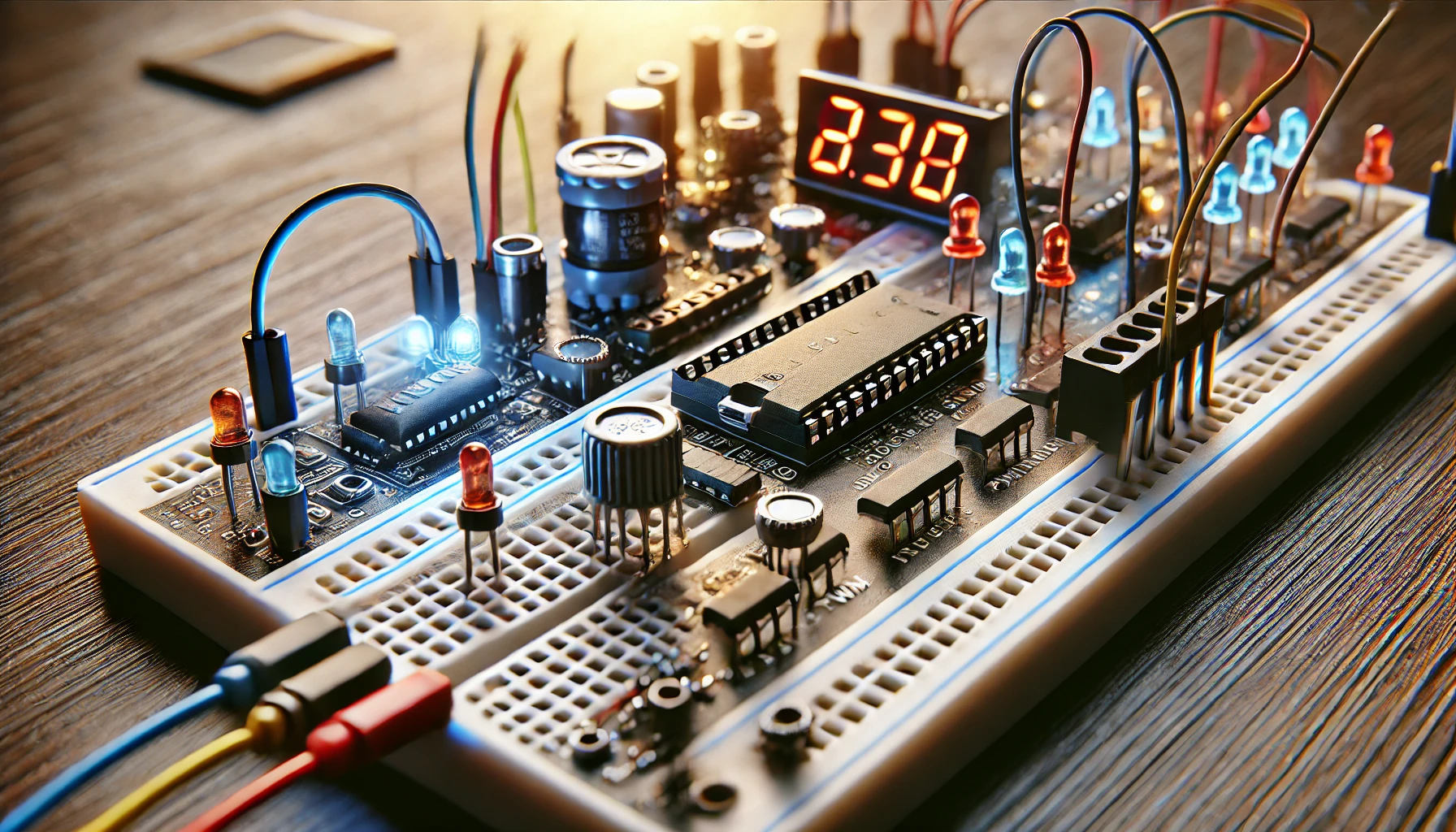
Mastering AVR Microcontroller Timers How to Generate Time Delays and PWM Signals , AVR microcontrollers, developed by Atmel (now part of Microchip Technology), are highly regarded in embedded systems for their simplicity, efficiency, and low cost. A critical feature of AVR microcontrollers is their built-in timers, which are fundamental for various time-dependent operations. Timers are used to generate time delays, create pulse-width modulation (PWM) signals, count external events, and more. This article dives deeper into AVR timers, exploring how they work and how you can utilize them in real-world applications.
Understanding Timers in AVR Microcontrollers
A timer is a hardware counter that increments based on a clock signal. In the context of AVR microcontrollers, timers are used for generating precise time intervals, producing PWM signals, counting events, and more. AVR microcontrollers are equipped with multiple timers, such as Timer/Counter 0, Timer/Counter 1, and Timer/Counter 2, each offering different features based on their bit resolution (8-bit or 16-bit).
Timers in AVR microcontrollers are often used for the following purposes:
- Time Delays: Creating precise and repeatable time delays.
- PWM Generation: Controlling devices like motors, LEDs, and audio signals.
- Event Counting: Counting the number of external pulses or events.
- Frequency Measurement: Measuring the frequency of external signals.
- Watchdog Timer: Ensuring the system resets if it malfunctions or enters an infinite loop.
Key Features of AVR Timers
- Prescaler: The prescaler divides the clock frequency, allowing the timer to increment more slowly. By adjusting the prescaler, you can change the range of time that the timer can measure.
- Mode of Operation: AVR timers support several modes, such as Normal mode, CTC (Clear Timer on Compare Match) mode, Fast PWM, Phase Correct PWM, etc.
- Interrupt Handling: Timers in AVR microcontrollers can trigger interrupts, allowing the CPU to perform other tasks while the timer counts in the background.
- Compare Match: Timers can compare their current count to a predefined value and take action (e.g., reset, toggle a pin, trigger an interrupt) when the count matches.
Types of Timers in AVR Microcontrollers
- Timer/Counter 0 (8-bit):
- Used for generating time delays, PWM signals, and event counting.
- Suitable for lower-resolution time-related operations.
- Supports Normal, CTC, and PWM modes.
- Timer/Counter 1 (16-bit):
- Provides higher resolution than Timer 0.
- Useful for generating longer time delays or high-frequency PWM signals.
- Supports Normal, CTC, Fast PWM, and Phase Correct PWM modes.
- Timer/Counter 2 (8-bit):
- Another 8-bit timer that can be used similarly to Timer 0.
- Typically used for low-precision applications like PWM generation.
Each of these timers can generate interrupts, allowing you to handle multiple tasks in parallel.
Using AVR Timers for Time Delay
One of the most common uses of AVR timers is to generate time delays. Timers allow you to pause the execution of code without blocking other tasks.
How Does Time Delay Work with Timers?
A timer counts clock pulses, and when it reaches a predetermined value, it triggers an event, such as setting a flag or interrupting the CPU. By adjusting the prescaler (which controls how fast the timer increments), you can create precise time delays without needing to rely on CPU cycles.
Example: Time Delay Using Timer 0
Consider an example where you want to generate a time delay of 1 millisecond using Timer 0:
- Select the Timer Mode: For simple delays, Timer 0 is typically used in Normal mode.
- Set the Prescaler: You select a prescaler value to adjust the timer’s counting rate.
- Set the Timer to Count: Start the timer and wait until it overflows or reaches the compare match value.
Here’s the code for generating a 1 ms delay using Timer 0 with an 8 MHz clock:
void timer0_delay_ms(uint16_t ms) {
// Set prescaler to 64 (this divides the 8 MHz clock by 64)
TCCR0 = (1<<CS01) | (1<<CS00);
for(uint16_t i = 0; i < ms; i++) {
TCNT0 = 0; // Reset the timer count
while ((TIFR0 & (1<<TOV0)) == 0); // Wait for overflow (TOV0 flag)
TIFR0 |= (1<<TOV0); // Clear the overflow flag
}
// Stop the timer
TCCR0 &= ~((1<<CS01) | (1<<CS00));
}
Explanation:
- Prescaler: The prescaler value of 64 means the timer increments slower, so it can generate a time delay based on the system clock.
- Overflow Check: The
TOV0
flag is set when Timer 0 overflows (reaches the maximum value). This flag is used to detect when a full timer cycle has been completed.
This code generates a delay in milliseconds by counting the number of timer overflows.
Using AVR Timers for PWM Generation
Pulse Width Modulation (PWM) is a widely used technique for controlling the power supplied to devices such as motors, LEDs, and audio equipment. AVR timers are perfect for generating PWM signals because they provide precise control over the duty cycle and frequency.
What is PWM?
PWM works by rapidly switching the output on and off at a fixed frequency, with the “on” time (duty cycle) being adjustable. This allows for effective control over the power delivered to a device.
PWM Modes in AVR
- Fast PWM Mode: In this mode, the timer counts from 0 to 255 (or 0 to 65535 for 16-bit timers) and resets when it reaches the maximum value. The duty cycle is controlled by changing the compare match value.
- Phase Correct PWM Mode: This mode generates a symmetrical waveform with a more gradual ramp-up and ramp-down. It’s used for smoother control of motors and other analog applications.
Example: Using Timer 1 for PWM Generation
Let’s look at an example where we generate a PWM signal with Timer 1 on an AVR microcontroller. We will generate a 50% duty cycle PWM signal.
void pwm_init() {
// Set Fast PWM mode (WGM = 14)
TCCR1A = (1 << COM1A1) | (1 << WGM10); // Non-inverted PWM on OC1A pin
TCCR1B = (1 << WGM12) | (1 << CS10); // No prescaler, Fast PWM mode
// Set the PWM frequency and duty cycle
OCR1A = 127; // 50% duty cycle (128/255)
}
void set_pwm_duty_cycle(uint8_t duty_cycle) {
OCR1A = (duty_cycle * 255) / 100; // Duty cycle range from 0 to 100%
}
Explanation:
- OCR1A: This register controls the duty cycle of the PWM signal. A value of 127 out of 255 corresponds to a 50% duty cycle.
- TCCR1A and TCCR1B: These registers control the mode of operation (Fast PWM) and the clock source (no prescaling in this case).
In this example, the set_pwm_duty_cycle
function can be used to change the duty cycle dynamically based on a 0-100% range.
Other Applications of AVR Timers
While time delays and PWM generation are two of the most common uses of timers, there are other important applications where AVR timers come in handy:
1. Event Counting
Timers in AVR can count external events (like pulses from sensors). For instance, you can use a timer to count the number of times a sensor is triggered within a given time frame.
2. Watchdog Timer (WDT)
The watchdog timer is a fail-safe mechanism that resets the microcontroller if it becomes unresponsive (e.g., due to a program crash or infinite loop). The WDT is periodically cleared by the program, and if it’s not cleared within a specific time frame, the system resets.
3. Frequency Measurement
AVR timers can measure the frequency of an external signal by capturing the time between two rising (or falling) edges of the signal. This is useful for applications like digital frequency counters.
Conclusion
AVR timers are versatile and powerful tools in embedded systems, allowing you to perform a wide range of tasks with minimal overhead. Whether you’re generating precise time delays, controlling PWM signals, counting events, or monitoring frequencies, understanding how to use these timers can significantly enhance the capabilities of your AVR-based projects.
By mastering the configuration of AVR timers, you can create more efficient and responsive systems. Experiment with different timer modes, prescalers, and compare values to better understand how to fine-tune your applications. With practice, AVR timers will become an invaluable part of your embedded system design toolkit.
Mastering AVR Microcontroller Timers How to Generate Time Delays and PWM Signals
Comments (0)