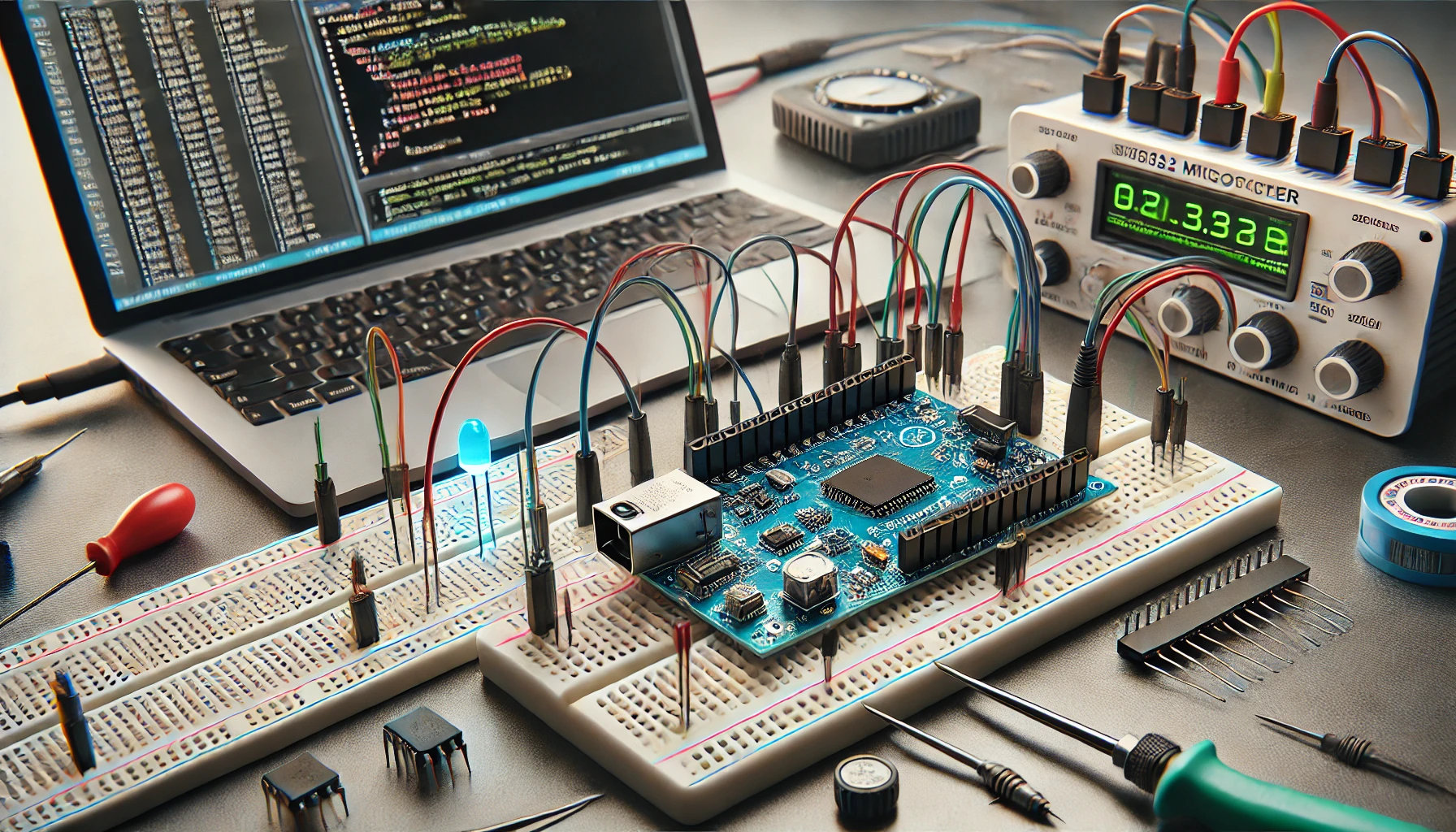
How to Configure GPIO Pins in STM32 Essential Tips for Input and Output Control , GPIO (General-Purpose Input/Output) pins are the workhorses of embedded systems. Whether you’re reading a sensor, controlling an LED, or communicating with external devices, GPIOs provide the necessary interfaces to interact with the physical world. For developers working with STM32 microcontrollers, mastering the configuration of GPIO pins is essential for building efficient, responsive systems.
This article delves into the process of configuring GPIO pins on STM32 microcontrollers, offering in-depth guidance on both input and output configurations. We will also include practical examples to solidify your understanding of how to effectively use GPIO pins for a variety of applications.
What Are GPIO Pins?
GPIO pins are flexible, multi-purpose pins on a microcontroller that can either receive data (as input) or send data (as output). These pins can be configured to work in different modes, such as digital input, digital output, analog input, or alternate functions like communication protocols (UART, SPI, I2C, etc.). STM32 microcontrollers provide a wide array of GPIO configuration options, making them incredibly versatile for various tasks.
Key Characteristics of GPIO Pins:
- Digital Input: Used to read a high or low signal, such as from a button or switch.
- Digital Output: Used to send a high or low signal, such as to drive an LED or relay.
- Analog Input: For sensors providing analog signals (like voltage levels).
- Alternate Function: GPIO pins can also be configured for specialized roles like UART, SPI, I2C, etc.
GPIO Configuration in STM32 Microcontrollers
STM32 microcontrollers offer a flexible approach to GPIO configuration, typically using two libraries: the HAL (Hardware Abstraction Layer) and the LL (Low-Layer) library. While HAL is more user-friendly and higher-level, LL gives you fine-grained control over hardware features. For most beginners, HAL is the recommended approach, as it simplifies the process and provides a large number of predefined functions.
1. Enabling the GPIO Port Clock
Before configuring any GPIO pin, you need to enable the clock for the GPIO port to which the pin belongs. In STM32, this is done through the RCC
(Reset and Clock Control) peripheral.
For example, if you want to configure a pin on port A (e.g., PA5), you would enable the clock for GPIOA first:
__HAL_RCC_GPIOA_CLK_ENABLE();
2. Configuring the Pin Mode
STM32 GPIO pins can be configured in different modes based on your application’s requirements. The basic modes are:
- Input: The pin reads digital signals (high or low).
- Output: The pin sends a digital signal.
- Analog: The pin is configured for analog signals (e.g., used for ADC input).
- Alternate Function: The pin is used for special functions like I2C, SPI, UART, etc.
To configure the mode of a pin, we use the GPIO_InitTypeDef
structure and the appropriate mode setting. Here’s an example of how to set PA5 as an output:
GPIO_InitTypeDef GPIO_InitStruct = {0};
GPIO_InitStruct.Pin = GPIO_PIN_5;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; // Push-pull output
GPIO_InitStruct.Pull = GPIO_NOPULL; // No pull-up/pull-down resistors
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; // Low speed
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
3. Setting Output Type
When configuring GPIO pins as outputs, you must specify whether the output will be push-pull or open-drain:
- Push-pull: A standard output that drives both high (Vcc) and low (GND) voltages.
- Open-drain: Only pulls the signal low, and an external pull-up resistor is required for driving the high state (common in I2C and certain logic applications).
Here’s how you can set the pin as push-pull (standard output):
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
If you wanted to configure it as an open-drain output:
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_OD;
4. Configuring Pin Speed
GPIO pins can operate at different speeds, which affects how quickly they change states. The available options are low, medium, high, and very high speeds.
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; // Set high speed for fast transitions
5. Pull-up and Pull-down Resistors
For input pins, it’s crucial to set the appropriate internal pull-up or pull-down resistors to avoid floating inputs. These internal resistors can be activated as either pull-up or pull-down based on your requirements.
- Pull-up: The pin is pulled high when no external signal is applied.
- Pull-down: The pin is pulled low when no external signal is applied.
Example of configuring a pin with an internal pull-up resistor:
GPIO_InitStruct.Pull = GPIO_PULLUP; // Activate pull-up resistor
If you don’t want any internal resistor:
GPIO_InitStruct.Pull = GPIO_NOPULL; // No pull-up/pull-down resistor
Practical Examples of GPIO Use
Example 1: Blinking an LED (GPIO as Output)
Let’s start with a simple example—blinking an LED connected to PA5. The following code demonstrates how to configure PA5 as an output and toggle it to blink an LED.
#include "stm32f4xx_hal.h"
void GPIO_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct = {0};
// Enable GPIOA clock
__HAL_RCC_GPIOA_CLK_ENABLE();
// Configure PA5 as output (push-pull)
GPIO_InitStruct.Pin = GPIO_PIN_5;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; // Push-pull mode
GPIO_InitStruct.Pull = GPIO_NOPULL; // No pull-up/pull-down
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; // Low speed
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
}
void Toggle_LED(void)
{
HAL_GPIO_TogglePin(GPIOA, GPIO_PIN_5); // Toggle LED state
}
int main(void)
{
HAL_Init(); // Initialize HAL Library
GPIO_Init(); // Configure GPIO
while (1)
{
Toggle_LED(); // Toggle the LED on PA5
HAL_Delay(500); // Delay for 500ms
}
}
Example 2: Reading a Button Press (GPIO as Input)
Next, let’s configure PA0 as an input to read a button press. When the button is pressed, the microcontroller can perform a specific action.
#include "stm32f4xx_hal.h"
void GPIO_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct = {0};
// Enable GPIOA clock
__HAL_RCC_GPIOA_CLK_ENABLE();
// Configure PA0 as input with pull-up resistor
GPIO_InitStruct.Pin = GPIO_PIN_0;
GPIO_InitStruct.Mode = GPIO_MODE_INPUT;
GPIO_InitStruct.Pull = GPIO_PULLUP; // Enable internal pull-up resistor
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
}
int main(void)
{
HAL_Init(); // Initialize HAL Library
GPIO_Init(); // Configure GPIO
while (1)
{
if (HAL_GPIO_ReadPin(GPIOA, GPIO_PIN_0) == GPIO_PIN_RESET) // Button is pressed
{
// Perform action, e.g., toggle LED
}
}
}
Example 3: Generating an Interrupt on GPIO Pin
STM32 allows you to configure GPIO pins to trigger an interrupt when the state of the pin changes (e.g., when a button is pressed). This is useful for creating responsive, interrupt-driven systems.
Here’s how you can set up a GPIO interrupt on PA0 to detect a falling edge (button press):
#include "stm32f4xx_hal.h"
void GPIO_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct = {0};
// Enable GPIOA clock
__HAL_RCC_GPIOA_CLK_ENABLE();
// Configure PA0 as input
GPIO_InitStruct.Pin = GPIO_PIN_0;
GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; // Interrupt on falling edge
GPIO_InitStruct.Pull = GPIO_PULLUP; // Pull-up resistor
HAL_GPIO_Init(GPIOA, &GPIO_InitStruct);
// Enable and configure EXTI line interrupt
HAL_NVIC_SetPriority(EXTI0_IRQn, 0, 0);
HAL_NVIC_EnableIRQ(EXTI0_IRQn);
}
// Interrupt handler
void EXTI0_IRQHandler(void)
{
if (__HAL_GPIO_EXTI_GET_IT(GPIO_PIN_0) != RESET)
{
__HAL_GPIO_EXTI_CLEAR_IT(GPIO_PIN_0);
// Handle button press event
}
}
Conclusion
The General-Purpose Input/Output (GPIO) pins on STM32 microcontrollers are versatile and essential for interacting with the outside world. Whether you’re controlling LEDs, reading sensors, or managing communication protocols, GPIO configuration forms the foundation of embedded system development.
By mastering the various configuration options—input, output, analog, and alternate function—you can make the most of your STM32 GPIO pins. The examples provided show you how to configure GPIOs for common tasks, from blinking an LED
How to Configure GPIO Pins in STM32 Essential Tips for Input and Output Control
Comments (0)